Swift의 프로토콜(Protocol) 사용 방법 총정리
Swift에서 프로토콜(Protocol)의 정의, 문법, 사용 방법을 알아보세요
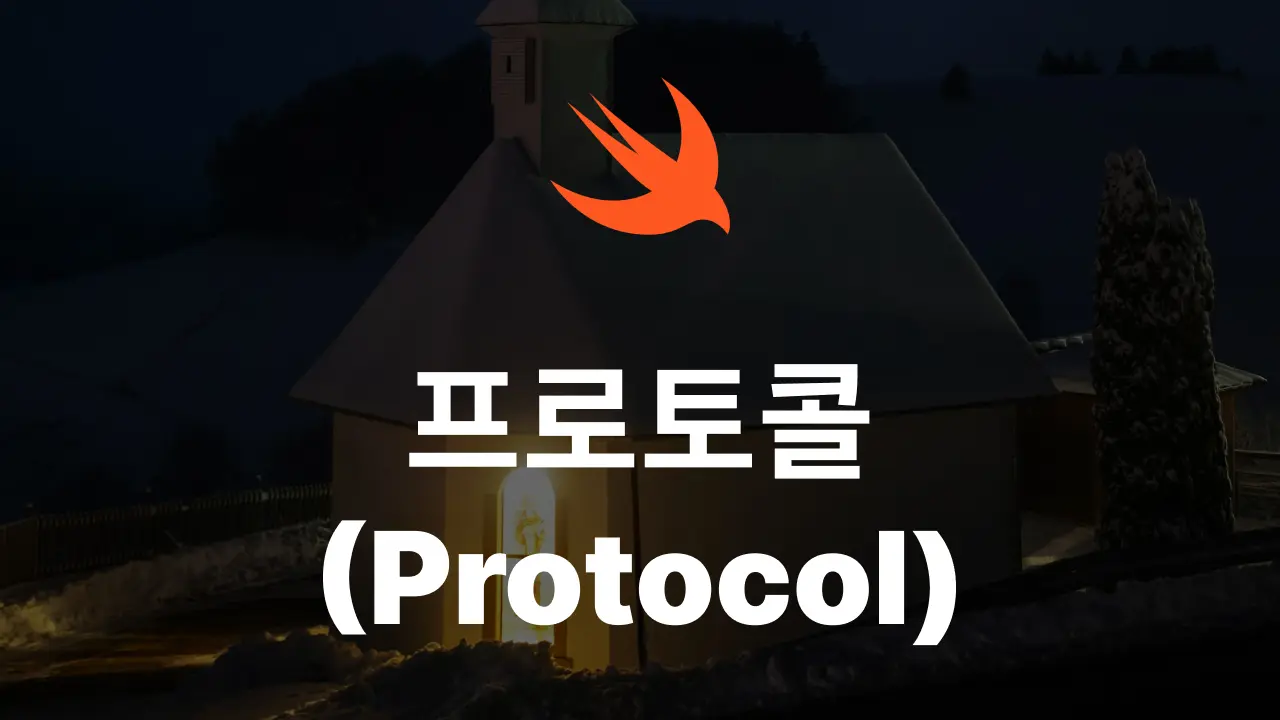
Swift에서 Protocol 란?
Swift에서 Protocol은 특정 역할을 수행하는 메서드나 프로퍼티의 집합을 정의하는 데 사용된다. Protocol은 클래스, 구조체, 열거형에 의해 구현될 수 있으며, 이를 통해 다양한 타입에 대한 공통 인터페이스를 정의할 수 있다.
Protocol의 기본구조
프로토콜은 protocol 키워드를 사용해 정의한다. 프로토콜은 속성이나 메서드에 대한 요구사항을 명시하며, 구현체는 프로토콜을 준수하기 위해 이러한 요구사항을 반드시 구현해야 한다.
protocol Greetable {
var name: String { get } // 읽기 가능한 속성 요구사항
func greet() // 메서드 요구사항
}
Protocol의 구현
프로토콜을 구현하는 타입은 프로토콜에 정의된 모든 요구사항을 구현해야 한다. 이를 통해 프로토콜을 준수하는 타입을 정의할 수 있다.
struct Person: Greetable {
var name: String
func greet() {
print("안녕하세요, \(name)님!")
}
}
let person = Person(name: "지수")
person.greet()
// 안녕하세요, 지수님!
Protocol의 속성 요구사항
속성 요구사항은 읽기/쓰기 속성을 정의할 수 있다. get
키워드는 읽기 가능한 속성을 나타내며, get set
은 읽기/쓰기 가능한 속성을 나타낸다.
protocol Describable {
var description: String { get } // 읽기 속성
var details: String { get set } // 읽기/쓰기 속성
}
struct Product: Describable {
var description: String
var details: String
}
var product = Product(description: "스마트폰", details: "최신 모델")
product.details = "최신 모델, 블랙 색상"
print(product.details)
// 최신 모델, 블랙 색상
Protocol의 메서드 요구사항
프로토콜은 메서드 요구사항을 정의할 수 있으며, 이를 통해 구현체가 특정 동작을 수행해야 함을 명시할 수 있다.
protocol Calculable {
func add(a: Int, b: Int) -> Int
func subtract(a: Int, b: Int) -> Int
}
struct Calculator: Calculable {
func add(a: Int, b: Int) -> Int {
return a + b
}
func subtract(a: Int, b: Int) -> Int {
return a - b
}
}
Protocol의 상속
프로토콜은 다른 프로토콜을 상속하여 새로운 요구사항을 추가할 수 있다. 이를 통해 기존 프로토콜을 확장하여 새로운 기능을 추가할 수 있다.
protocol Identifiable {
var id: String { get }
}
protocol Verifiable: Identifiable {
func verify() -> Bool
}
struct User: Verifiable {
var id: String
init(id: String) {
self.id = id
}
func verify() -> Bool {
return id.count > 5
}
}
let user = User(id: "SwiftUser")
print(user.verify())
// true