Swift Dictionary(딕셔너리)의 사용방법
Swift의 Dictionary(딕셔너리)의 사용방법을 배워보세요.
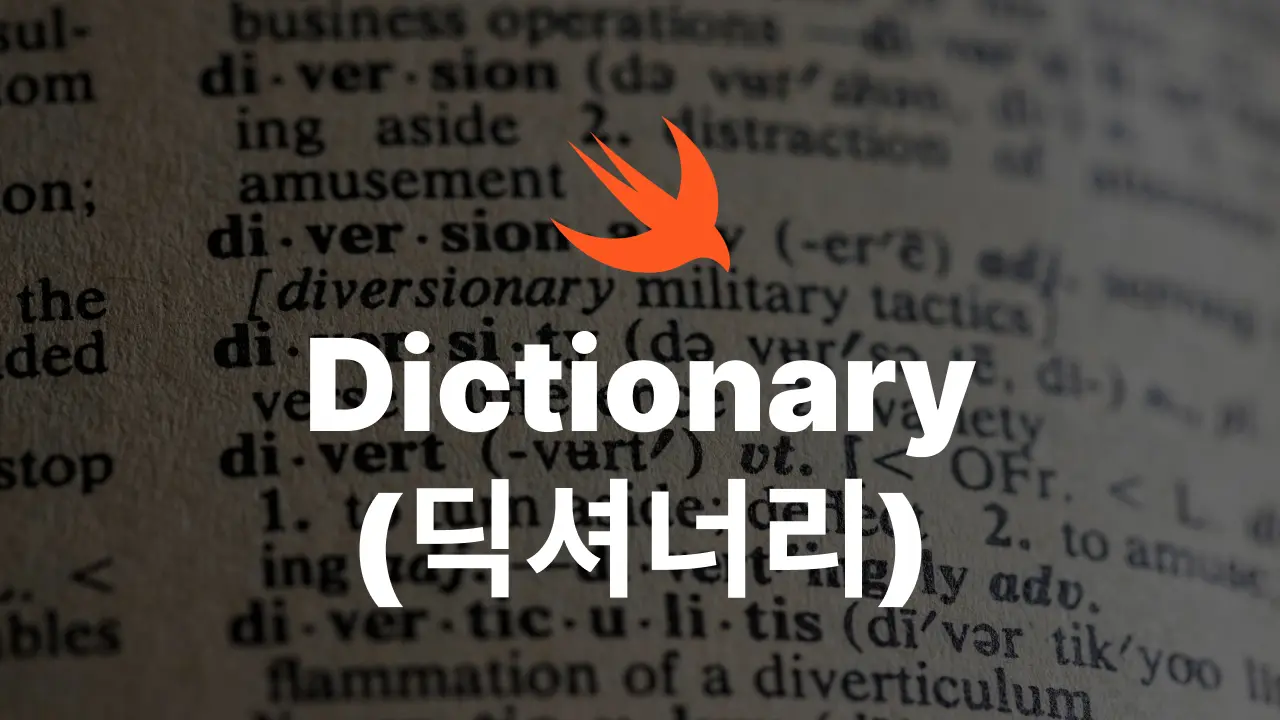
Swift에서 Dictionary(딕셔너리) 란?
Swift에서 Dictionary는 키-값 쌍으로 데이터를 저장하는 컬렉션 타입입니다. 배열처럼 순차적으로 데이터가 저장되는 것이 아니라, 키를 통해 값에 빠르게 접근할 수 있는 구조입니다. 키는 고유해야 하며, 각 키는 하나의 값과 연결된니다.
1. Dictionary 생성
var dictionary: Dictionary<String, Int> = ["apple": 1, "banana": 2, "cherry": 3]
var dictionary: [String: Int] = ["apple": 1, "banana": 2, "cherry": 3]
2. 빈 Dictionary 생성
var emptyDictionary: [String: Int] = [:]
var emptyDictionary = [String: Int]()
3. Dictionary 요소 접근
Dictionary에서 키를 사용해서 값을 가져올 때는 값이 없을 수도 있으므로 ??
연산자를 사용해서 기본값을 설정해주는 것이 좋습니다.
let appleValue = dictionary["apple"]
print(appleValue ?? "Not found) // Optional(1)
4. Dictionary 요소 추가
순서는 보장되지 않습니다.
dictionary["grape"] = 4
print(dictionary) // ["apple": 1, "banana": 2, "cherry": 3, "grape": 4]
5. Dictionary 요소 삭제
dictionary.removeValue(forKey: "banana")
print(dictionary) // ["apple": 1, "cherry": 3, "grape": 4]
6. Dictionary 요소 업데이트
dictionary["apple"] = 10
print(dictionary) // ["apple": 10, "banana": 2, "cherry": 3, "grape": 4]
dictionary.updateValue(11, forKey: "grape")
print(dictionary) // ["apple": 10, "banana": 2, "cherry": 3, "grape": 11]
7. Dictionary가 요소를 포함하는지 확인
var dictionary: [String: Int] = ["apple": 1, "banana": 2, "cherry": 3, "grape": 4]
let containsBanana = dictionary.contains(where: { $0.key == "banana" })
print(containsBanana) // true
8. Dictionary 요소 반복
var dictionary: [String: Int] = ["apple": 10, "cherry": 3, "grape": 4]
for (key, value) in dictionary {
print("\(key): \(value)")
}
// cherry: 3
// grape: 4
// apple: 10
9. Dictionary 항목 수
var dictionary: [String: Int] = ["apple": 1, "banana": 2, "cherry": 3, "grape": 4]
print(dictionary.count) // 4
10. Key 배열과 Value 배열로 부터 Dictionary 생성
let keys = ["apple", "banana", "cherry"]
let values = [1, 2, 3]
let dictionary = Dictionary(uniqueKeysWithValues: zip(keys, values))
print(dictionary) // ["apple": 1, "banana": 2, "cherry": 3]
11. Dictionary 병합
let dictionary1: [String: Int] = ["apple": 1, "banana": 2]
let dictionary2: [String: Int] = ["cherry": 3, "grape": 4]
let mergedDictionary = dictionary1.merging(dictionary2) { $1 } // 중복되는 키가 있으면 뒤에 있는 값을 사용합니다.
print(mergedDictionary) // ["apple": 1, "banana": 2, "cherry": 3, "grape": 4]