Swift 데이터 타입
Swift 데이터 타입에 대해서 알아보자.
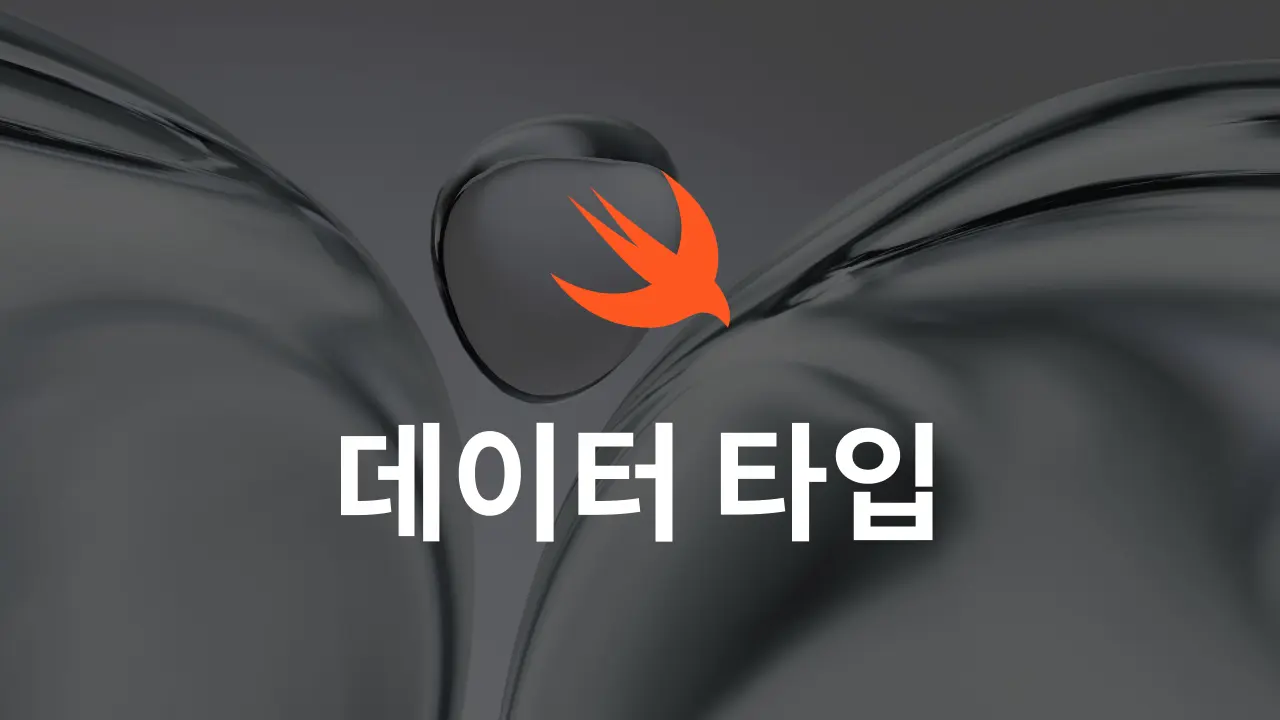
Swift 데이터 타입
Swift에서 사용할 수 있는 데이터 타입은 다음과 같다.
- Int
- UInt
- Float
- Double
- Bool
- Character
- String
- Array
- Set
- Dictionary
- Tuple
Int
정수 타입으로 음수, 양수 모두 표현 가능하다.
var num1: Int = 10
print(num1) // 10
let maxInt: Int = Int.max
let minInt: Int = Int.min
print(maxInt) // 9223372036854775807
print(minInt) // -9223372036854775808
// 메모리상에서 얼마나 많은 사이즈를 차지하는지 확인
MemoryLayout.size(ofValue: num1) // 8 (Byte) = 64 (Bit)
// 숫자가 크지 않다면 Int8을 사용해서 메모리 사용량을 줄일 수 있다.
var smallInt: Int8 = 10
MemoryLayout.size(ofValue: smallInt) // 1 (Byte) = 8 (Bit)
// Int8의 최대값, 최소값
let smallMaxInt: Int8 = Int8.max
let smallMinInt: Int8 = Int8.min
print(smallMaxInt) // 127
print(smallMinInt) // -128
// Int는 기본적으로 Int64이며 다음과 같은 Int 타입들이 있다.
// Int8, Int16, Int32, Int64 = Int
UInt
정수 중 양수만 표현 가능하다.
var num1: UInt = 10
print(num1) // 10
// UInt의 최대값, 최소값
let maxUInt: UInt = UInt.max
let minUInt: UInt = UInt.min
print(maxUInt) // 18446744073709551615
print(minUInt) // 0
// 메모리상에서 얼마나 많은 사이즈를 차지하는지 확인
MemoryLayout.size(ofValue: num1) // 8 (Byte) = 64 (Bit)
// UInt는 기본적으로 UInt64이며 UInt8, UInt16, UInt32, UInt64 타입이 있다.
Float
부동소수점 타입으로 소수점 이하 6~7자리까지 표현 가능하다.
let pi: Float = 3.14159
print(pi) // 3.14159
// Float의 최대값, 최소값
let maxFloat: Float = Float.greatestFiniteMagnitude
let minFloat: Float = -Float.greatestFiniteMagnitude
print(maxFloat) // 3.4028235e+38
print(minFloat) // -3.4028235e+38
// 메모리상에서 얼마나 많은 사이즈를 차지하는지 확인
MemoryLayout.size(ofValue: pi) // 4 (Byte) = 32 (Bit)
MemoryLayout<Float>.size // 4 (Byte) = 32 (Bit)
Double
부동소수점 타입으로 소수점 이하 15자리까지 표현 가능하다.
let pi: Double = 3.141592
print(pi) // 3.141592
// Double의 최대값, 최소값
let maxDouble: Double = Double.greatestFiniteMagnitude
let minDouble: Double = -Double.greatestFiniteMagnitude
print(maxDouble) // 1.7976931348623157e+308
print(minDouble) // -1.7976931348623157e+308
// 타입 추론
let root2 = 1.4142135623730951
print(type(of: root2)) // Double
Bool
불리언 타입으로 true, false 두 가지 값만 표현 가능하다.
let isSwiftFun: Bool = true
let isJavaDifficult: Bool = false
print(isSwiftFun) // true
print(isJavaDifficult) // false
if isSwiftFun {
print("Swift is fun!")
} else {
print("Java is difficult!")
}
Character
문자 타입으로 하나의 문자를 표현 가능하다.
var char1: Character = "A"
print(char1) // A
String
문자열 타입으로 문자열을 표현 가능하다.
var str1: String = "Hello, Swift!"
print(str1) // Hello, Swift!
Array
배열 타입으로 여러 개의 값을 순서대로 저장하고 관리할 수 있다.
var arr1: Array<Int> = [1, 2, 3]
var arr2: [Int] = [1, 2, 3]
print(arr1) // [1, 2, 3]
print(arr2) // [1, 2, 3]
Set
집합 타입으로 중복되지 않는 유니크한 값을 저장하고 관리할 수 있다.
var uniqueNumbers: Set<Int> = [1, 2, 3, 3, 4]
var uniqueNumbers2 = Set([1, 2, 3, 3, 4]) // 타입 추론
print(uniqueNumbers) // {1, 2, 3, 4}
print(uniqueNumbers2) // {1, 2, 3, 4}
Dictionary
키-값 쌍으로 데이터를 저장하고 관리할 수 있다.
var dict1: Dictionary<String, Int> = ["one": 1, "two": 2, "three": 3]
var dict2: [String: Int] = ["one": 1, "two": 2, "three": 3]
var capitals: [String: String] = ["Korea": "Seoul", "Japan": "Tokyo"]
capitals["USA"] = "Washington D.C."
print(capitals) // ["Korea": "Seoul", "Japan": "Tokyo", "USA": "Washington D.C."]
Tuple
튜플 타입으로 여러 개의 값을 하나로 묶어서 관리할 수 있다.
var person: (name: String, age: Int, isStudent: Bool) = ("John", 25, true)
print(person) // ("John", 25, true)
// 튜플의 타입 확인
print(type(of: person)) // (String, Int, Bool)
// 튜플의 요소에 접근
print(person.name) // John
print(person.age) // 25
print(person.isStudent) // true
print(person.0) // John
print(person.1) // 25
print(person.2) // true
// 튜플 분해
let (name, age, isStudent) = person
// 보간법(interpolation) 사용해서 출력
print("\(name) is \(age) years old. He is \(isStudent ? "a student" : "not a student").") // John is 25 years old. He is a student.